Playing around with GraphQL
I started playing around a bit with GraphQL in a rails environment. And to be honest, the documentation around this stuff can be a bit difficult to follow.
I started by building out a standard rails api project and setting up all the docker dependencies. Got a very basic data object built out with some CRUD scaffolding and then decided to plug in GraphQL.
I followed the instructions at https://github.com/rmosolgo/graphql-ruby and got up and running pretty quickly. The API documentation for building queries is pretty simple. However, I'm not sure I'm quite there on scalability of the code.
module Types
class QueryType < Types::BaseObject
graphql_name 'Query'
field :projects, [ProjectType], null: false do
description "Projects"
#argument :id, ID, required: true
end
def projects
Project.all
end
end
end
Scaling the query schema
All of your queries get put into a single query_type.rb file. While it's not large in this example, I can see it potentially get huge after I add more and more read queries. Which led me to look on github for other ways to do this. I found this...
A really neat but lengthy query schema. The closest solution I found was the following:
# app/graphql/types/query_type.rb
module Types
QueryType = GraphQL::ObjectType.new.tap do |root_type|
# class MutationType < Types::BaseObject
# Add root-level fields here.
root_type.name = 'QueryType'
root_type.description = 'The query root'
root_type.interfaces = []
root_type.fields = Util::FieldCombiner.combine([
QueryTypes::InvestorQueryType,
QueryTypes::MeetingQueryType
])
# They will be entry points for queries on your schema.
end
end
How to query GraphQL
The next roadblock was how do I query this from an external app? I had originally tried to test this in Postman but eventually found the amazing Insomnia
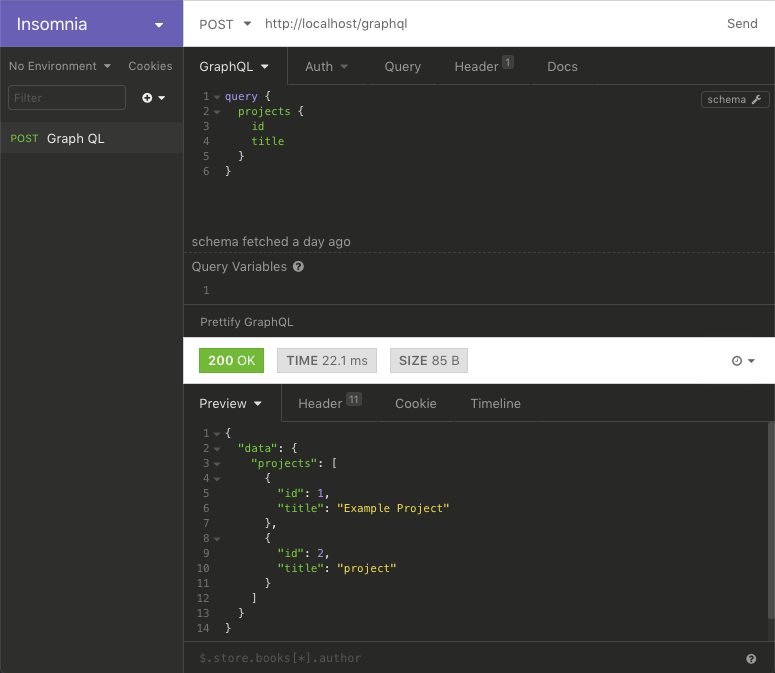
I also probably fought building mutations for maybe 3 hours. The docs on this are probably the worst for someone getting started. I eventually resorted to using one of the rake generators for mutations so that I could move forward a little bit. I think that I will have similar issues with code scalability.
Conclusions
My first round conclusions are a bit foggy. I think I need to play around a bit more, however I'm starting to think that rails might not be the best format for GraphQL. A framework on top of a framework that seems a bit heavy for much of the CRUD operations that GraphQL would handle.