Naming Things for Programmers
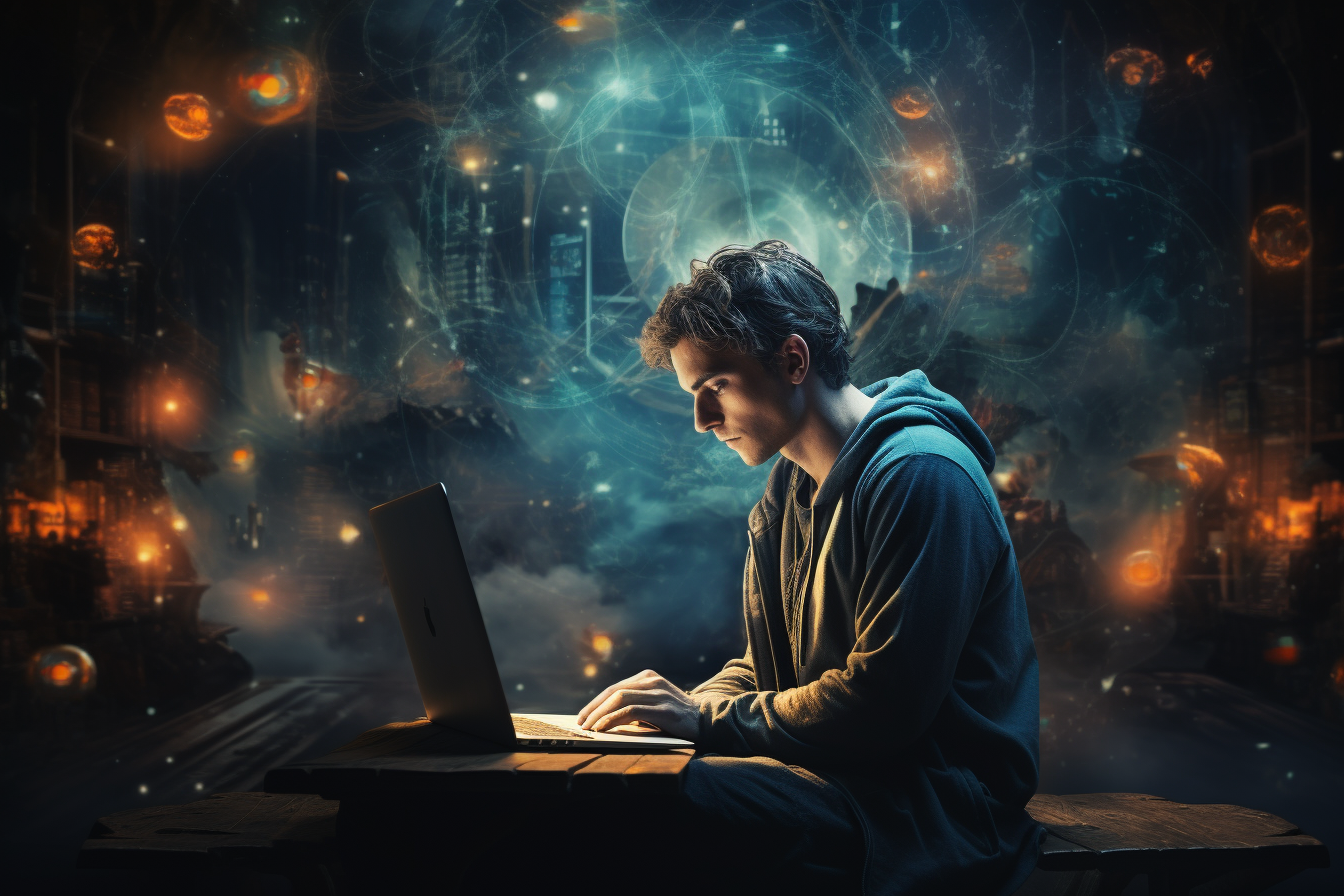
The art of naming things is no easy task. That being said, I would argue it might be one of the most important skills, if not THE most important skill a programmer learns. While there is no hard and fast rule on how to go about naming things, hopefully these tips will help you get better at it.
Be Consistent
Often conventions are setup specifically for the purpose of consistency across multiple developers. Controllers and tables are plural while entities are singular. Do we use pascal case, snake case, camel case? It doesn't really matter, but once you choose your conventions be consistent across the project.
Be Meaningful
Make sure that your names have meaning and are not just some filler or placeholder name. I should be able to read the name of your variable, function, or file and have a pretty good idea of what it is used for. If you have to comment to explain your variable or function, then the name could probably be more meaningful.
Be Positive
This could also be titled, avoid double negatives. By making your variables positive, you achieve this goal.
var disabled = true;
// hard to read
if( !disabled ) {
// do things
}
var enabled = false;
// easier to read
if( !enabled ) {
// do things
}
Use Pronounceable Names
Programming is often a team sport, so use names that are easily pronounceable to make your code easier to read by others.
Avoid Hard To Name Variables, Functions, and Files
If the functionality or variable you are trying to name is too long or hard to name, there is a good chance that it needs to be broken down into smaller easier to name chunks.
Avoid Generic Terms
Avoid terms like helper, manager, utils, service, etc. These terms give no context as to how to use the item in question. They also tend to be generic buckets to just dump functions without any context or organization. They are the messy junk room of code.
Use Searchable Names
When we get thrown into new code, the first thing we tend to do is search for things. If we use shorthand names that are not easily searchable, then we hamper readability and remove a powerful tool from our coding tool belt.
Refactor Confusing Names
Don't be afraid to refactor confusing names. Most IDEs are able to quickly rename a variable, class, function, or file and update all the references.